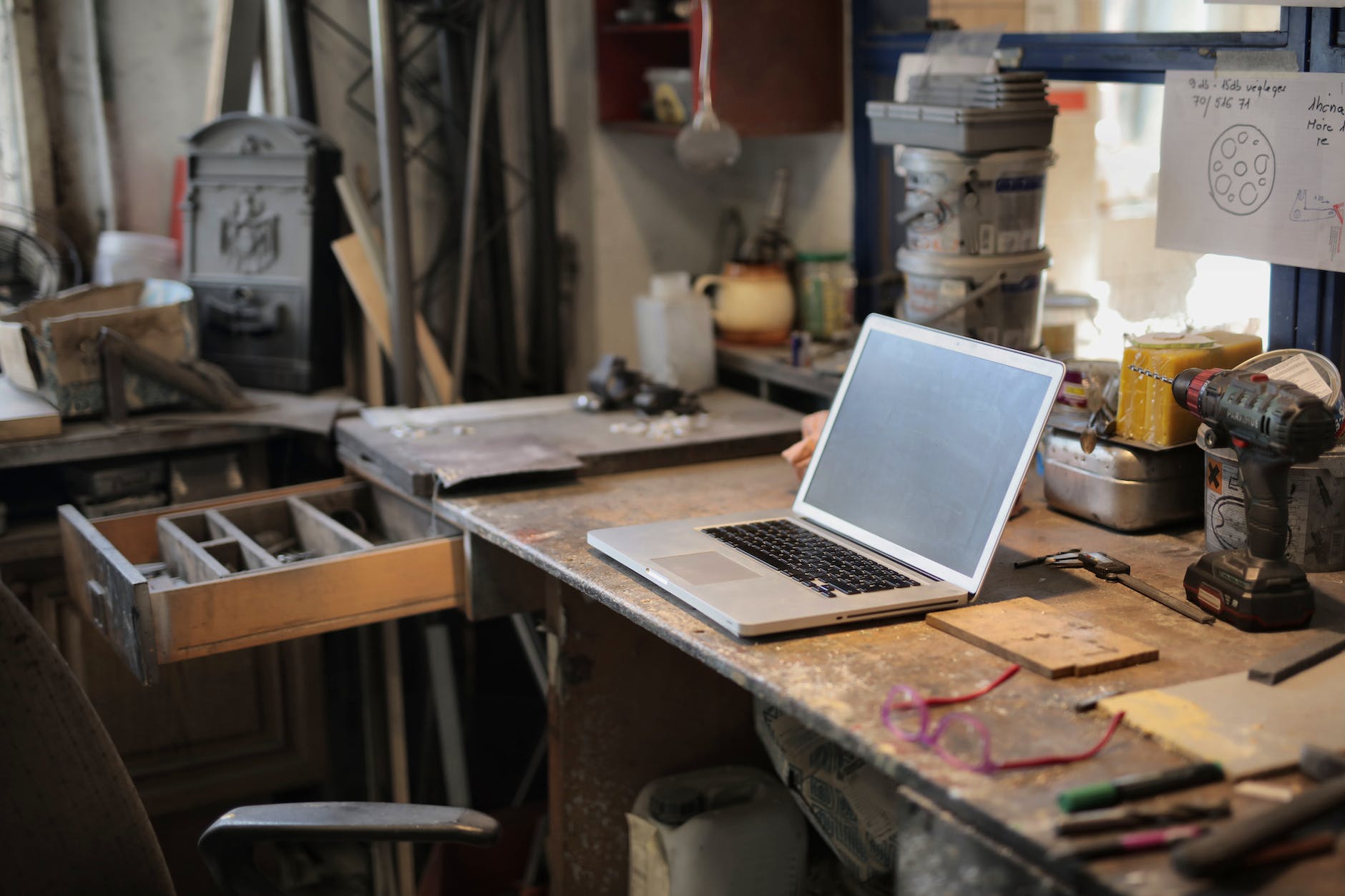
Understanding Visual Regression Testing and Getting Started with Puppeteer
Visual regression testing ensures web application’s visual consistency by comparing screenshots of different versions. Maintaining a uniform appearance is vital for user experience and brand integrity. Enter Puppeteer, a potent Node.js library by Google that helps in automation testing. It captures screenshots, enabling easy comparison to detect unintended visual changes. In this guide, we’ll dive into harnessing Puppeteer’s capabilities for effective visual regression testing.
In the dynamic realm of web development, maintaining visual consistency across different versions of a website is a significant challenge. Visual glitches, though seemingly minor, can lead to user frustration, diminished brand credibility, and even loss of revenue. This is where visual regression testing comes into play – a process designed to identify unintended visual changes between different iterations of a web application. In this comprehensive guide, we’ll delve into the concept of visual regression testing, its importance, and how to initiate the process using Puppeteer, a powerful Node.js library developed by Google.
Understanding Visual Regression Testing
Defining Visual Regression Testing: Visual regression testing is a quality assurance technique used to identify discrepancies in the visual appearance of a web application between different versions. Unlike traditional functional testing that focuses on the functionality of the application, visual regression testing concentrates on the visual aspect. It involves capturing screenshots of specific pages or components of a website and then comparing them against a baseline reference to detect any unintended visual changes.
Importance of Visual Consistency: In today’s digital landscape, users expect a seamless and consistent experience across various devices and browsers. Even small visual discrepancies, such as misaligned elements, color variations, or font inconsistencies, can negatively impact the user experience. Maintaining visual consistency is essential for brand identity, user trust, and ultimately the success of the web application. A simple visual bug, if left unnoticed, could lead to a negative perception among users and drive them away from the site.
Real-world Examples: Visual bugs have caused notable disruptions in various industries. For instance, an e-commerce website’s checkout button shifting position during an update could confuse users and hinder the purchase process. Similarly, a banking app displaying irregular font sizes might raise concerns about the application’s security. The infamous example of LinkedIn’s unintended color change causing uproar among users is a testament to how visual discrepancies can trigger strong reactions and undermine user confidence.
Introducing Puppeteer
Overview of Puppeteer: Puppeteer is an open-source Node.js library developed by Google that provides a high-level API for controlling headless Chrome or Chromium browsers. It offers a plethora of features for automating browser interactions, web scraping, generating PDFs, and, importantly, conducting visual regression testing. Puppeteer allows developers to navigate and manipulate web pages just like a user would, making it a versatile tool for various web automation tasks.
Programmatic Control of Browsers: With Puppeteer, developers can programmatically open web pages, simulate user interactions (such as clicks and form submissions), and extract information from the pages’ DOM (Document Object Model). The “headless” mode allows browsers to operate without a graphical user interface, enabling automated testing without any visible browser window.
Flexibility and Automation Capabilities: Puppeteer’s flexibility extends beyond just testing. It can be used for tasks like generating screenshots for documentation, monitoring websites for changes, and automating repetitive tasks like filling out forms. This makes it a valuable addition to a developer’s toolkit, saving time and ensuring accuracy.
Setting Up Your Environment
Installing Node.js: Before diving into Puppeteer, it’s essential to have Node.js installed on your system. If you haven’t already done so, you can download and install Node.js from the official Node.js website (https://nodejs.org/). Node.js provides the runtime environment for executing JavaScript on the server side.
Setting Up a Node.js Project: Once Node.js is installed, you can create a new project directory where you’ll initiate your Puppeteer-based visual regression testing. Open your terminal, navigate to the desired directory, and run the following commands:
mkdir my-visual-regression-project
cd my-visual-regression-project
npm init -y
The above commands create a new directory, navigate into it, and initialize a new Node.js project with default settings using the -y flag.
Installing Puppeteer: To use Puppeteer in your project, you need to install it as a dependency. Run the following command in your project directory:
npm install puppeteer
This command installs Puppeteer and its dependencies within your project.
Creating Your First Puppeteer Script
Importing Puppeteer and Launching a Browser Instance: In your Node.js project, create a new JavaScript file (e.g., index.js) and import Puppeteer at the beginning of the file:
const puppeteer = require(‘puppeteer’);
Next, let’s launch a browser instance using Puppeteer:
(async () => {
const browser = await puppeteer.launch();
// Rest of your code will go here
})();
The puppeteer.launch() function initiates a headless browser instance. The use of an asynchronous function (async () => {…}) allows you to use the await keyword within the function.
Opening a Web Page and Taking a Screenshot: To showcase the basics of Puppeteer and lay the foundation for visual regression testing, let’s open a web page and capture a screenshot:
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘https://www.example.com’);
await page.screenshot({ path: ‘example.png’ });
await browser.close();
})();
In this code snippet, we create a new page using browser.newPage(), navigate to a URL using page.goto(), capture a screenshot using page.screenshot(), and then close the browser instance using browser.close().
Building Blocks for Visual Regression Testing: These fundamental steps – launching a browser, navigating to a webpage, and capturing a screenshot – form the building blocks for visual regression testing using Puppeteer. The next sections will delve deeper into creating a workflow for effective visual regression testing.
Understanding Visual Regression Workflow
Workflow Overview: A typical visual regression testing workflow involves the following steps:
- Capture Baseline Screenshots: The initial version of the web application serves as the baseline. Capture screenshots of various pages or components that need to be tested for visual consistency.
- Make Code Changes: Implement code changes, updates, or improvements in the web application.
- Capture New Screenshots: After making changes, capture new screenshots of the same pages or components.
- Compare Screenshots: Compare the new screenshots with the baseline screenshots to identify any visual differences or regressions.
- Analyze and Address Differences: Analyze the differences and determine whether they are intended changes or unintended regressions. Address any unintended visual changes.
Taking a Baseline Screenshot: A baseline screenshot serves as the reference for future comparisons. To capture baseline screenshots using Puppeteer, you would follow similar steps as shown earlier. However, this time you’ll store the captured screenshots in a designated folder or directory. It’s advisable to organize your screenshots in a structured manner, such as by page or component.
Capturing Subsequent Screenshots: Once code changes are made and updates are applied, you’ll need to capture new screenshots for comparison. Puppeteer allows you to navigate to the same pages and capture new screenshots using the same methods as before. The key is consistency – ensure that you capture screenshots under the same conditions (browser viewport size, device emulation settings, etc.) as the baseline screenshots.
Comparing Screenshots: To identify visual differences, you’ll need
to compare the new screenshots with the baseline screenshots. This process involves pixel-by-pixel comparison to detect even the slightest variations. While manual comparison is possible for smaller projects, it quickly becomes impractical as the application’s complexity grows. This is where Puppeteer’s image comparison capabilities come in handy.
Implementing Visual Regression Testing with Puppeteer
Writing a Basic Visual Regression Test: Let’s dive into writing a basic visual regression test using Puppeteer. In this example, we’ll create a simple test to capture screenshots of a webpage and compare them to a baseline.
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Navigate to the webpage you want to test
await page.goto(‘https://www.example.com’);
// Capture a screenshot and save it as the baseline
await page.screenshot({ path: ‘baseline.png’ });
// Apply code changes or updates to the webpage
// Navigate to the same webpage after making changes
await page.goto(‘https://www.example.com’);
// Capture a new screenshot for comparison
await page.screenshot({ path: ‘newScreenshot.png’ });
await browser.close();
})();
In this script, we first capture a screenshot of the initial version of the webpage and save it as the baseline. After making code changes, we navigate to the same webpage, capture a new screenshot, and save it for comparison.
Using “Golden” Images: To simplify comparison, a common technique is to use “golden” images – baseline images that represent the expected visual appearance. Instead of pixel-by-pixel comparison, you compare the new screenshot against the golden image. This method reduces false positives caused by minor layout changes or anti-aliasing variations.
To use golden images, save the baseline screenshot as a reference and then compare the new screenshots against it. Puppeteer provides the necessary functions for image comparison, making the process straightforward.
Handling Visual Differences
Using Puppeteer’s Image Comparison Features: Puppeteer provides built-in capabilities for image comparison, making it efficient to detect visual differences. By capturing screenshots of the baseline and updated versions of your web application, you can use Puppeteer’s pixelDiff() function to compare these images pixel by pixel. This function highlights the areas of the images that differ, aiding in identifying specific visual changes.
Importance of Tolerance Settings: Visual discrepancies might not always be errors; they can stem from minor layout changes or anti-aliasing variations. Puppeteer allows you to set tolerance levels for image comparison, enabling you to define an acceptable range of differences. Tolerance settings accommodate slight deviations and prevent false positives triggered by insignificant changes.
Scenarios with Acceptable Differences: In certain scenarios, visual differences are acceptable due to dynamic content. For example, a weather widget displaying real-time data may show variations in temperature or conditions. In such cases, setting a tolerance level that accommodates these changes while flagging significant deviations ensures accurate testing without generating unnecessary alerts.
Integrating Puppeteer Tests into CI/CD
Significance of CI/CD Integration: Integrating visual regression tests into your Continuous Integration and Continuous Deployment (CI/CD) pipeline enhances the development workflow. Automated visual regression testing alongside other tests ensures that new code changes do not introduce unexpected visual regressions, maintaining the application’s visual integrity.
Setting Up Automated Puppeteer Tests: Popular CI/CD tools like Jenkins, Travis CI, and CircleCI can be leveraged to automate Puppeteer tests. Start by configuring your CI/CD environment to run a specific script that executes your Puppeteer-based visual regression tests. This script should trigger on each code change or deployment, ensuring that visual regressions are caught early in the development process.
Best Practices for Effective Visual Regression Testing
Maintaining a Successful Strategy:
- Test Coverage: Select critical pages and components for testing to maximize efficiency.
- Regular Execution: Run visual regression tests as part of your development routine, ideally after each significant change.
- Version Control: Store baseline screenshots in version control to track changes over time.
- Team Collaboration: Involve designers, developers, and QA teams to collectively identify and address visual regressions.
Managing False Positives and Negatives:
- Tolerance Settings: Fine-tune tolerance levels to avoid false positives for minor changes.
- Baseline Updates: Regularly update baseline screenshots to reflect intentional visual changes.
- Automated Reporting: Implement automated reporting to flag false positives and investigate false negatives.
Regular Test Maintenance and Baseline Updates:
- Scheduled Reviews: Plan regular reviews of baseline screenshots to verify their accuracy.
- Updating Baselines: Update baselines when design changes are implemented intentionally to ensure accurate comparisons.
- Documentation: Maintain clear documentation of your visual regression testing process for new team members.
Advanced Topics and Further Resources
Testing Responsive Designs: Extend your visual regression testing to cover responsive design by testing across various device sizes and orientations.
User Interaction Testing: Explore Puppeteer’s capabilities to simulate user interactions, enabling testing of dynamic visual changes triggered by user actions.
Additional Resources and Documentation:
- Puppeteer’s Official Documentation: https://pptr.dev/
- GitHub Repository: https://github.com/puppeteer/puppeteer
- Tutorials on Advanced Puppeteer Usage: Online tutorials and blog posts offer insights into more complex use cases and strategies.
Elevating Visual Regression Testing with LambdaTest
In the realm of visual regression testing, LambdaTest stands out as an advanced tool. Powered by Visual AI technology, LambdaTest intelligently detects visual discrepancies, even within dynamic content. Seamlessly integrating with Puppeteer, LambdaTest streamlines the testing process by offering benefits such as cross-browser and cross-device testing, batch testing, and effortless CI/CD integration. By harnessing the combined power of Puppeteer and LambdaTest, developers can efficiently manage baselines, identify differences, and ensure a visually consistent user experience. LambdaTest proves to be an invaluable asset for enhancing visual regression testing strategies.
Conclusion
In the ever-evolving digital landscape, maintaining visual consistency across web applications is crucial for user satisfaction and brand identity. Visual regression testing using Puppeteer empowers developers to automate the process of detecting unintended visual changes. By harnessing Puppeteer’s capabilities, setting up a CI/CD pipeline, and following best practices, teams can ensure that their applications deliver a seamless and visually appealing experience to users. Embrace the power of Puppeteer and explore the world of visual regression testing to create web applications that truly shine. Remember, the journey toward impeccable visual consistency begins with a single automated test.